[C#] Struct VS Object.
15/11/23 - #csharp #softwareengineering #struct #object
In the realm of C# programming, the concepts of struct and object are fundamental. Though both are used for creating custom types, they exhibit significant differences that impact performance, memory usage, and code behavior. This article delves into these differences to provide programmers with a clear guide on when and how to use each.
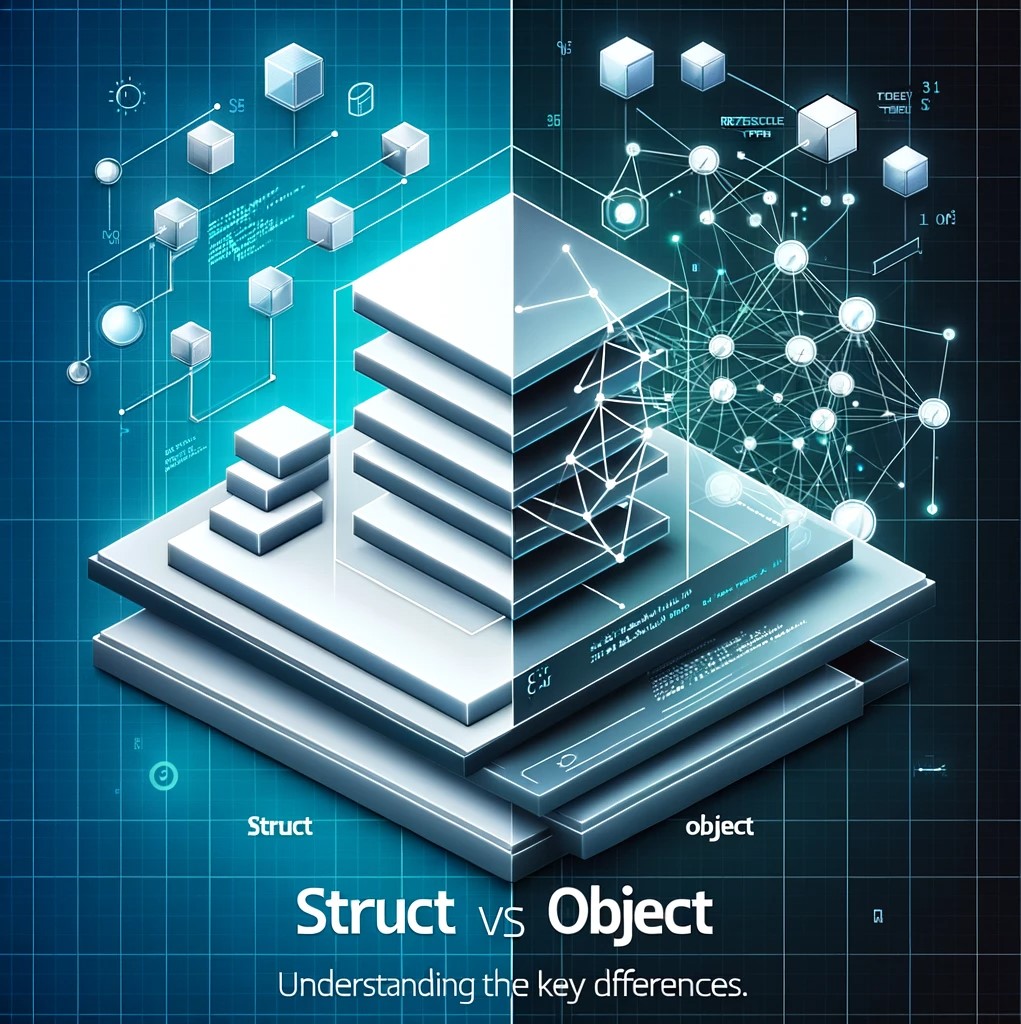
Definitions and Characteristics
Struct
- Semantics: A struct in C# is a value type. This means when it is assigned to a new variable or passed to a method, a copy of the original value is made.
- Memory Usage: struct is generally more memory-efficient for small data types. They are allocated on the stack, which is quicker in allocation and deallocation.
- Limitations: They do not support inheritance, except for interface implementation, and cannot have a parameterless constructor.
Object
- Semantics: An object in C# is a reference type. This means when an object is assigned to a new variable, both references point to the same object in memory.
- Memory Usage: Objects are allocated in the heap, requiring more complex memory management with a garbage collector.
- Flexibility: They fully support inheritance and polymorphism, allowing for greater flexibility and code reuse.
Practical Comparison
Performance
- Struct can be faster for small data types as they avoid the overhead of garbage collection. However, passing large structures can be less efficient due to data copying.
- Object might be slower in allocation, but offer advantages in sharing and manipulating complex data.
Usage Scenarios
- Use struct for small, immutable types like numeric types, DateTime, or custom tuples.
- Use object for modeling complex entities and object hierarchies, as in object-oriented programming.
Choosing between struct and object in C# should not be based merely on stylistic preferences, but on a thoughtful evaluation of the program's performance needs, design, and memory usage. Understanding the differences between these two types is crucial for writing efficient and maintainable code.